45 tick size matplotlib
Major and minor ticks — Matplotlib 3.4.3 documentation import matplotlib.pyplot as plt import numpy as np from matplotlib.ticker import (MultipleLocator, AutoMinorLocator) t = np. arange (0.0, 100.0, 0.1) s = np. sin (0.1 * np. pi * t) * np. exp (-t * 0.01) fig, ax = plt. subplots ax. plot (t, s) # Make a plot with major ticks that are multiples of 20 and minor ticks that # are multiples of 5. How to Adjust Marker Size in Matplotlib? - GeeksforGeeks scatter is a method present in matplotlib library which is used to set individual point sizes. It takes 3 parameters 2 data points and a list of marker point sizes. Python3 import matplotlib.pyplot as plt data1 = [1, 2, 3, 4, 5] data2 = [0, 0, 0, 0, 0] sizes = [10, 20, 30, 40, 50] plt.scatter (data1, data2, sizes) plt.xlabel ('x-axis')
Changing the tick size in Matplotlib - SkyTowner local_offer Python Matplotlib. To change the tick size in Matplotlib, use the tick_params (~) method: plt.tick_params(axis="both", labelsize=15) plt.plot( [1,2,3]) plt.show() filter_none. The output is as follows: To change only the tick size of only either the x-axis or the y-axis: plt.tick_params(axis="x", labelsize=15) # To change the x-axis.
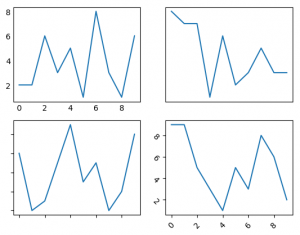
Tick size matplotlib
Change Tick Frequency in Matplotlib - Stack Abuse x and y range from 0-50, and the length of these arrays is 100. This means, we'll have 100 datapoints for each of them. Then, we just plot this data onto the Axes object and show it via the PyPlot instance plt: Now, the frequency of the ticks on the X-axis is 20. Set Tick Labels Font Size in Matplotlib | Delft Stack plt.xticks(fontsize= ) to Set Matplotlib Tick Labels Font Size from matplotlib import pyplot as plt from datetime import datetime, timedelta xvalues = range(10) yvalues = xvalues fig,ax = plt.subplots() plt.plot(xvalues, yvalues) plt.xticks(fontsize=16) plt.grid(True) plt.show() plt.xticks(fontsize=16) 如何在 Matplotlib 中设置刻度标签 xticks 字体大小 | D栈 - Delft Stack 在这里, fontsize 设置刻度标签的字体大小。. from matplotlib import pyplot as plt from datetime import datetime, timedelta import numpy as np xvalues = np.arange(10) yvalues = xvalues fig,ax = plt.subplots() plt.plot(xvalues, yvalues) plt.xticks(xvalues) ax.set_xticklabels(xvalues, fontsize=16) plt.grid(True) plt.show()
Tick size matplotlib. Change plot size in Matplotlib - Python - GeeksforGeeks Here are various ways to change the default plot size as per our required dimensions or resize a given plot. Method 1: Using set_figheight () and set_figwidth () For changing height and width of a plot set_figheight and set_figwidth are used Python3 import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [1, 2, 3, 4, 5] plt.xlabel ('x - axis') How to Set Tick Labels Font Size in Matplotlib (With Examples) How to Set Tick Labels Font Size in Matplotlib (With Examples) You can use the following syntax to set the tick labels font size of plots in Matplotlib: import matplotlib.pyplot as plt #set tick labels font size for both axes plt.tick_params(axis='both', which='major', labelsize=20) #set tick labels font size for x-axis only plt.tick_params(axis='x', which='major', labelsize=20) #set tick labels font size for y-axis only plt.tick_params(axis='y', which='major', labelsize=20) Adjust Tick Label Size Matplotlib With Code Examples To change only the size of the label: How do I increase the tick size in Matlab? Direct link to this answer If you want your tick to be longer and thicker, you can increase both the length AND thickness of the tick marks with the TickLength and LineWidth properties of the axes. ax. TickLength = [k, k]; % Make tick marks longer. ax.31-Dec-2015 Change the label size and tick label size of colorbar using Matplotlib ... # Change the tick label size of color-bar. im.figure.axes[1].tick_params(axis="", labelsize=21) axis = x, y or both. labelsize = int; Example 1: In this example, we are changing the label size in Plotly Express with the help of method im.figure.axes[0].tick_params(axis="both", labelsize=21), by passing the parameters axis value as both axis and label size as 21.
Ticks Font Size Matplotlib With Code Examples - folkstalk.com plt.xticks (fontsize=14, rotation=90) There are a variety of approaches that can be taken to solve the same problem Ticks Font Size Matplotlib. The remaining solutions are discussed further down. ax.tick_params (axis='both', which='major', labelsize=10) Matplotlib xticks() in Python With Examples - Python Pool The matplotlib.pyplot.xticks () function is used to get or set the current tick locations and labels of the x-axis. It passes no arguments to return the current values without modifying them. Before we look into various implementations of Matplotlib xticks (), let me brief you with the syntax and return the same. Syntax of Matplotlib xticks () How to Change the Number of Ticks in Matplotlib - Statology You can use the following syntax to change the number of ticks on each axis in Matplotlib: #specify number of ticks on x-axis plt.locator_params(axis='x', nbins=4) #specify number of ticks on y-axis plt.locator_params(axis='y', nbins=2) How to Set Axis Ticks in Matplotlib (With Examples) - Statology You can use the following basic syntax to set the axis ticks in a Matplotlib plot: #set x-axis ticks (step size=2) plt. xticks (np. arange (min(x), max(x)+1, 2)) ... By default, Matplotlib has chosen to use a step size of 2.5 on the x-axis and 5 on the y-axis.
matplotlib.axes.Axes.tick_params — Matplotlib 3.6.0 documentation Tick label font size in points or as a string (e.g., 'large'). labelcolor color. Tick label color. colors color. Tick color and label color. zorder float. ... Examples using matplotlib.axes.Axes.tick_params # Scatter plot with histograms. Scatter plot with histograms. Creating annotated heatmaps. Creating annotated heatmaps. Image Masked. How to Change the Number of Ticks in Matplotlib? So, we can use this function to control the number of ticks on the plots. syntax: matplotlib.pyplot.locator_params(axis='both', tight=None, nbins=None **kwargs) Parameter: axis - The axis we need to change the number of ticks or tighten them. tight - Takes in a bool value weather the ticks should be tightened or not matplotlib.ticker — Matplotlib 3.6.0 documentation class matplotlib.ticker.AsinhLocator(linear_width, numticks=11, symthresh=0.2, base=10, subs=None) [source] # Bases: Locator An axis tick locator specialized for the inverse-sinh scale This is very unlikely to have any use beyond the AsinhScale class. Note This API is provisional and may be revised in the future based on early user feedback. Python Matplotlib Tick_params + 29 Examples - Python Guides Read Python plot multiple lines using Matplotlib. Matplotlib tick_params font size. In this section, we'll learn how to change the font size of the tick labels in Matplotlib tick_params. The labelsize argument is used to change the font size of the labels.. The following is the syntax for changing the font size of the label:
Tick formatters — Matplotlib 3.6.0 documentation MultipleLocator (0.25)) ax. xaxis. set_ticks_position ('bottom') ax. tick_params (which = 'major', width = 1.00, length = 5) ax. tick_params (which = 'minor', width = 0.75, length = 2.5, labelsize = 10) ax. set_xlim (0, 5) ax. set_ylim (0, 1) ax. text (0.0, 0.2, title, transform = ax. transAxes, fontsize = 14, fontname = 'Monospace', color = 'tab:blue')
How to Set Tick Labels Font Size in Matplotlib? - GeeksforGeeks Plot a graph on data using matplotlib. Change the font size of tick labels. (this can be done by different methods) To change the font size of tick labels, any of three different methods in contrast with the above mentioned steps can be employed. These three methods are: fontsize in plt.xticks/plt.yticks ()
如何在 Matplotlib 中设置刻度标签 xticks 字体大小 | D栈 - Delft Stack 在这里, fontsize 设置刻度标签的字体大小。. from matplotlib import pyplot as plt from datetime import datetime, timedelta import numpy as np xvalues = np.arange(10) yvalues = xvalues fig,ax = plt.subplots() plt.plot(xvalues, yvalues) plt.xticks(xvalues) ax.set_xticklabels(xvalues, fontsize=16) plt.grid(True) plt.show()
Set Tick Labels Font Size in Matplotlib | Delft Stack plt.xticks(fontsize= ) to Set Matplotlib Tick Labels Font Size from matplotlib import pyplot as plt from datetime import datetime, timedelta xvalues = range(10) yvalues = xvalues fig,ax = plt.subplots() plt.plot(xvalues, yvalues) plt.xticks(fontsize=16) plt.grid(True) plt.show() plt.xticks(fontsize=16)
Change Tick Frequency in Matplotlib - Stack Abuse x and y range from 0-50, and the length of these arrays is 100. This means, we'll have 100 datapoints for each of them. Then, we just plot this data onto the Axes object and show it via the PyPlot instance plt: Now, the frequency of the ticks on the X-axis is 20.
Post a Comment for "45 tick size matplotlib"